We are using networking in mobile applications from a while. In the early, we used main thread for network call but Google stop calls network request on main thread when Google released the Honeycomb version. After that we have many libraries for networking calls.
Introduction
This is because network requests are used to run long tasks that required more processing power. So making network calls on the main thread result to the NetworkOnMainThreadException error in higher SDK levels. We can not also run network request on UI thread .This is because if we run any long task on the UI thread than it can “freeze” a user’s interface. To solve these problems we have different classes and libraries like AsyncTask, Retrofit, Volley, and others to carry out networking in mobile applications.
Volley Library in Android
Volley is an HTTP kind of library that is mostly used for caching and making a network request in Android applications. It make us easy for manage the processing and caching of network requests and help developers to save there valuable time from writing the same network call/cache code. For volley library it is very complex for doing large download or streaming operations because volley holds all responses in memory during parsing request.
Features of Volley:
- Volley allow us to request queuing and prioritization.
- Volley provide us effective request cache and memory management mechanism
- Volley is an extensibility and customization of the library to our needs
- Volley provide us mechanism of cancelling the requests
Advantages of using Volley:
- All the task that has to be done in android with Networking , can be done with the use of Volley.
- Volley provide automatic scheduling of network requests.
- Volley has catching mechanism
- Volley provide multiple concurrent network connections.
- Volley has ability for cancelling request API.
- we can prioritization of request in volley.
Disadvantages of Volley
- It’s not capable for doing streaming and large downloads.
- It has 5 sec of connection time by default
- It’s slower in performance as compared to Retrofit.
- It’s has a complex code structure as compare to Retrofit
Classes used in Volley
- Request: This class of volley contains the necessary information to make network API requests.
- Request Queue: This class is use to dispatch network API requests..
Useful Terminology
- Volley – an HTTP library which is used for caching and making a network API request in Android applications.
- API -it is software that contains data which is used for communication between two applications.
- JSON – (JavaScript Object Notation) is a data-interchange format which is lightweight .
- Permission It is a statements that allow an android application to access different properties that contain a user’s sensitive information.
Prerequisites
- Android Studio has to be installed.
- A basic knowledge about APIs and how to work with making Requests.
- A basic knowledge of how to use REST APIs, JSON, and making Requests.
- A basic knowledge and understanding of XML and Java programming language.
Why Volley?
- Volley can pretty much do everything with Networking in Android that has to do .
- Volley has ability to schedules all network requests automatically .
- Volley provides transparent disk and memory caching.
- Volley provides powerful customization abilities.
- Volley provides debugging and tracing tools.
Volley Uses Caches To Improve Performance In Android:
Volley also uses caches concept which help us to improve the performance of App. For example, lets say to fetch image from server and description from a JSON array created in server API by the using Asynctask. lets assume this content is fetched in the portrait mode and now when user rotate screen and change it to landscape mode. Then the activity is destroyed and the content will be fetched again due to creation of activity after destroyed.
Since the user is calling the same network request again to server so it is a a poor user experience and also waste of resource .Volley solve this problem as it caches the data. When user request again to fetch the same data from the server, it will not call request again from serve it provide data from cache while help in improving user experience.
Below is the basic diagram of Volley:
In first image as you can see its fetch information from server and later save it into caches. When user wants to fetch same information again it does not fetch from server it fetches from caches as you can see in image second.
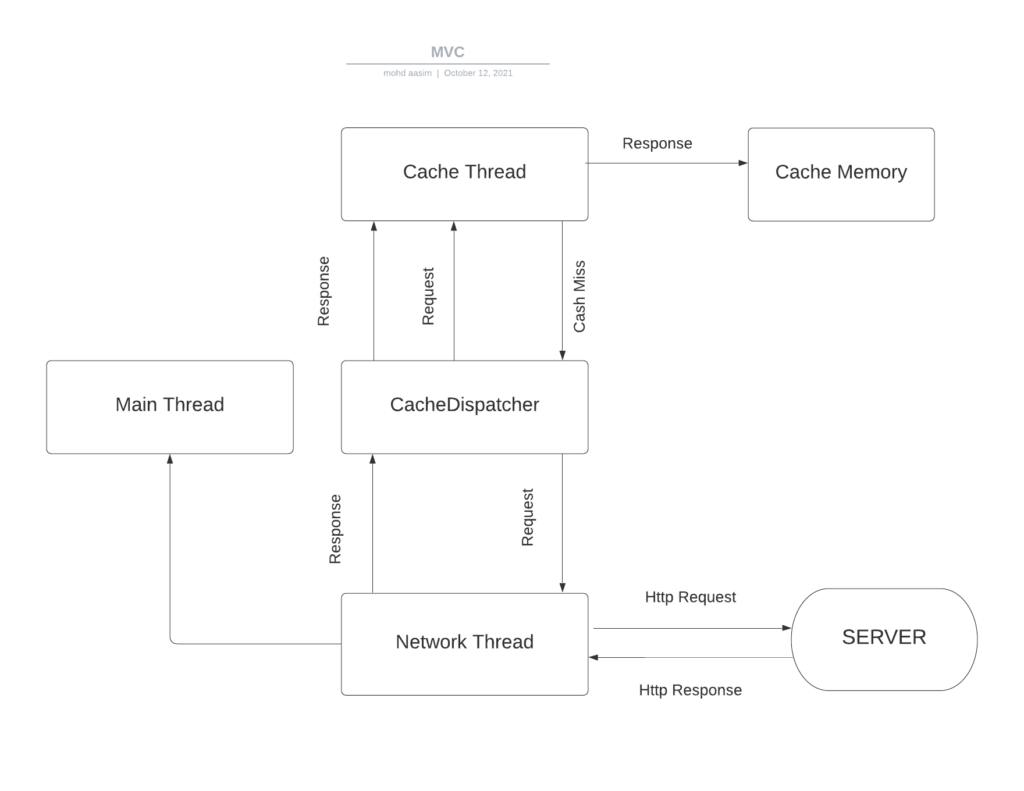
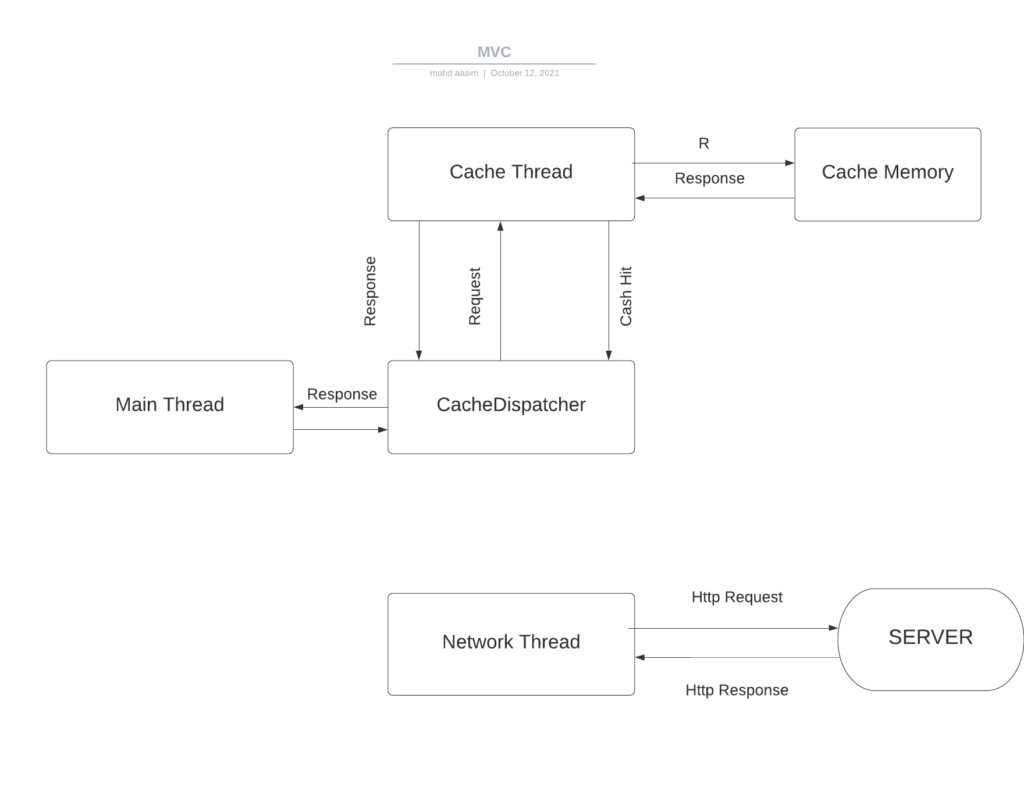
How to Import Volley and add Permissions:
To start working with volley first we need to import gradle dependency of volley then we need to add internet permission in manifest file as follows:-
1. Create new project.
2. add the following dependency:
3. dependencies{
4. //...
5. implementation 'com.android.volley:volley:1.0.0'
6. }
7.
8. In AndroidManifest.xml add the internet permission:
9. <uses-permission
10. android:name="android.permission.INTERNET />"
Types of Request using Volley Library:–
1:-String Request
String url = "https:// string_url/";
StringRequest stringRequest = new StringRequest(Request.Method.GET,url,
new Response.Listener() {
@Override
public void onResponse(String response)
{
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error)
{
}
});
requestQueue.add(stringRequest);
2:-JSONObject Request
String url = "https:// json_url/";
JsonObjectRequest jsonObjectRequest = new JsonObjectRequest(Request.Method.GET,url,null,
new Response.Listener() {
@Override
public void onResponse(JSONObject response)
{
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error)
{
}
});
requestQueue.add(jsonObjectRequest);
3:-JSONArray Request
JsonArrayRequest jsonArrayRequest = new JsonArrayRequest(Request.Method.GET,url,null,
new Response.Listener() {
@Override
public void onResponse(JSONArray response)
{
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error)
{
}
});
requestQueue.add(jsonArrayRequest);
4:-Image Request
int max - width = ...;
int max_height = ...;
String URL = "http:// image_url.png";
ImageRequest imageRequest = new ImageRequest(URL,
new Response.Listener() {
@Override
public void
onResponse(Bitmap response)
{
// Assign the response
// to an ImageView
ImageView imageView= (ImageView)findViewById(R.id.imageView);
imageView.setImageBitmap(response);
}
},
max_width, max_height, null);
requestQueue.add(imageRequest);
5:-Adding Post Parameters
String tag_json_obj = "json_obj_req";
String
url = "https:// api.xyz.info/volley/person_object.json";
ProgressDialog pDialog = new ProgressDialog(this);
pDialog.setMessage("Loading...PLease wait");
pDialog.show();
JsonObjectRequest jsonObjReq = new JsonObjectRequest(Method.POST,url,null,
new Response.Listener() {
@Override
public void onResponse(JSONObject response)
{
Log.d(TAG, response.toString());
pDialog.hide();
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error)
{
VolleyLog.d(TAG, "Error: "+ error.getMessage());
pDialog.hide();
}
}) {
@Override
protected Map getParams()
{
Map params = new HashMap();
params.put("name", "Androidhive");
params.put("email", "abc@androidhive.info");
params.put("password", "password123");
return params;
}
};
AppController.getInstance().addToRequestQueue(jsonObjReq, tag_json_obj);
6:-Adding Request Headers
String tag_json_obj = "json_obj_req";
String url= "https:// api.androidhive.info/volley/person_object.json";
ProgressDialog pDialog = new ProgressDialog(this);
pDialog.setMessage("Loading...");
pDialog.show();
JsonObjectRequest
jsonObjReq= new JsonObjectRequest(Method.POST,url,null,
new Response.Listener() {
@Override
public void onResponse(JSONObject response)
{
Log.d(TAG, response.toString());
pDialog.hide();
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error)
{
VolleyLog.d(TAG, "Error: "+ error.getMessage());
pDialog.hide();
}
}) {
@Override
public Map getHeaders() throws AuthFailureError
{
HashMap headers = new HashMap();
headers.put("Content-Type", "application/json");
headers.put("apiKey", "xxxxxxxxxxxxxxx");
return headers;
}
};
AppController.getInstance().addToRequestQueue(jsonObjReq, tag_json_obj);
7:-Handling the Volley Cache
// Loading request from cache
Cache cache = AppController.getInstance().getRequestQueue().getCache();
Entry entry = cache.get(url);
if (entry != null) {
try {
String data= new String(entry.data, "UTF-8");
// handle data, like converting it
// to xml, json, bitmap etc.,
}
catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
}
}
else
{
// If cached response doesn't exists
}
// Invalidate cache
AppController.getInstance().getRequestQueue().getCache().invalidate(url, true);
// Turning off cache
// String request
StringRequest stringReq= new StringRequest(....);
// disable cache
stringReq.setShouldCache(false);
// Deleting cache for particular cache</strong>
AppController.getInstance().getRequestQueue().getCache().remove(url);
// Deleting all the cache
AppController.getInstance().getRequestQueue().getCache().clear(url);
8:-Cancelling Request
// Cancel single request
String tag_json_arry = "json_req";
ApplicationController.getInstance().getRequestQueue().cancelAll("feed_request");
// Cancel all request
ApplicationController.getInstance().getRequestQueue().cancelAll();
9:-Request Prioritization
private Priority priority = Priority.HIGH;
StringRequest strReq = new StringRequest(Method.GET,Const.URL_STRING_REQ,
new Response.Listener() {
@Override
public void onResponse(String response) {
Log.d(TAG, response.toString());
msgResponse.setText(response.toString());
hideProgressDialog();
} },
new Response.ErrorListener() {
@Override
public void
onErrorResponse(VolleyError error) {
VolleyLog.d(TAG,"Error: "+ error.getMessage());
hideProgressDialog();
} }) {
@Override
public Priority getPriority()
{
return priority;
}
};
Conclusion
In this article we have why we use volley for network call in android, what is volley library ,what are the advantages of volley, disadvantages of volley, features of volley, class of volley ,Terminology of volley and why we should use volley. We have also discuss type of request in volley and how can we implements these request in volley.